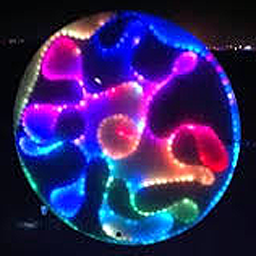 |
FastLED 3.9.7
|
Loading...
Searching...
No Matches
2#include "./led_layout_array.h"
6 static const int kLedRepeatTable[] = {
96 const int* out = kLedRepeatTable;
97 const int size =
sizeof(kLedRepeatTable) /
sizeof(*kLedRepeatTable);
104 static const int kLedRepeatTable[] = {
194 const int* out = kLedRepeatTable;
195 const int size =
sizeof(kLedRepeatTable) /
sizeof(*kLedRepeatTable);
201 return LedCurtinArray();
203 return LedLuminescentArray();